Login/ Register with firebase
Today I am going to login/register using firebase.
But, before moving forward we need to create project in firebase console. After creating project in firebase console then follow the below steps.
Lets first build our app with expo.
expo init loginWithFirebase
It creates a new project name loginWithFirebase and cd to this project
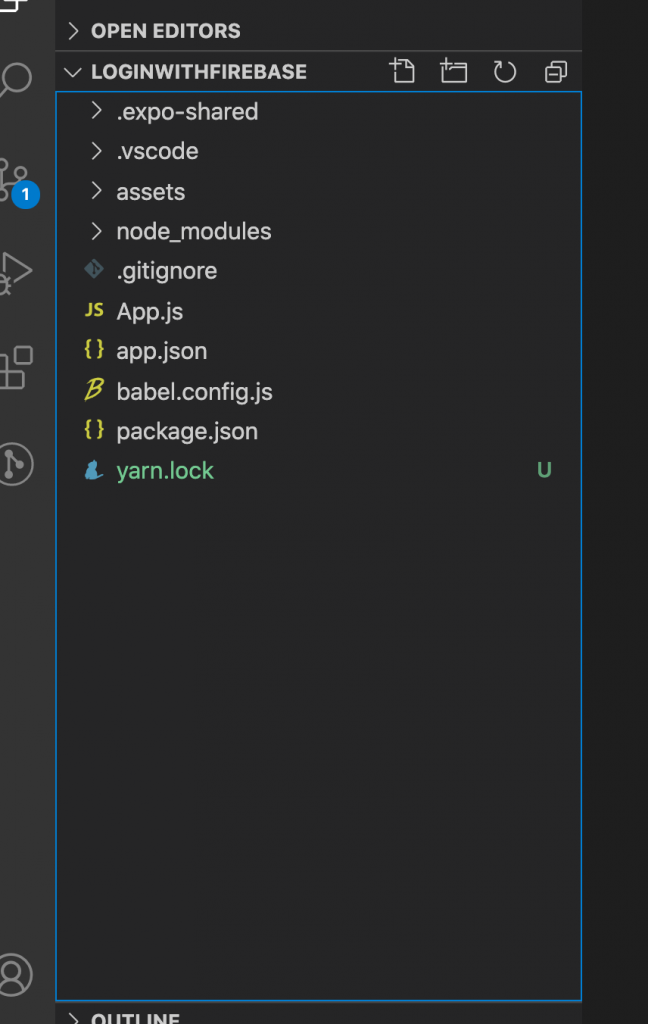
We will have the above folder structure.
Now, we need to install the dependencies required for our project
expo install firebase
expo install react-native-gesture-handler react-native-reanimated react-native-screens react-native-safe-area-context @react-native-community/masked-view
Now, let’s create a folder named src and constant. Inside it create a file name as Navigation.js, Entry.js, Home.js, and inside constant let’s create a file name as constant.js where we will put all the firebase configuration.
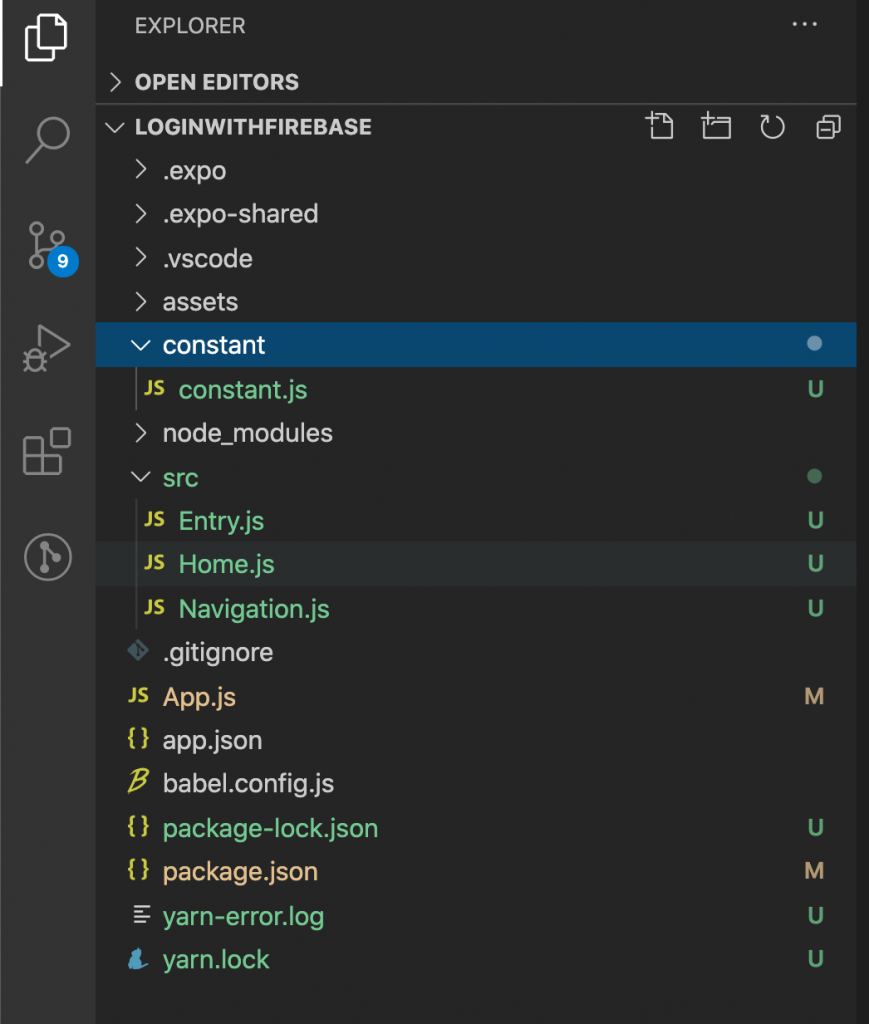
Now, Lets go the App.js file and write the following code.
import React from "react";
import { View, Text } from "react-native";
import Navigation from "./src/Navigation";
export default function App() {
return (
<View style={{ flex: 1 }}>
<Navigation />
</View>
);
}
Here, We are importing the Navigation file, and returning it.
In the Navigation.js file, we are importing our Entry.js and Home.js file, which are in the stack navigator.
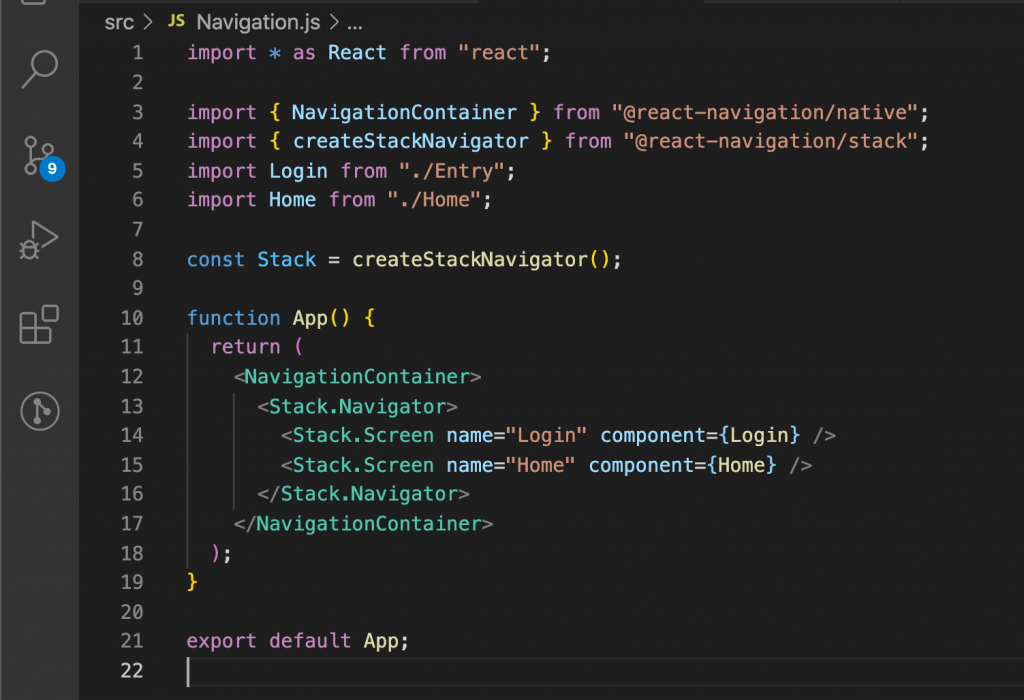
Now, lets write our code for the login and register in Entry.js file.
import React, { useState, useEffect } from "react";
import {
StyleSheet,
Text,
View,
TouchableOpacity,
TextInput,
} from "react-native";
import { auth } from "../constant/constant";
export default function App({ navigation }) {
const [email, setEmail] = useState("");
const [password, setPassword] = useState("");
useEffect(() => {
const subscribe = auth.onAuthStateChanged((authuser) => {
if (authuser) {
navigation.navigate("Home");
}
});
return subscribe; // unsubscribe on unmount
}, []);
const handleChangeEmail = (val) => {
setEmail(val);
};
const handleChangePassword = (val) => {
setPassword(val);
};
const handleLoginPress = () => {
auth.onAuthStateChanged((authuser) => {
if (authuser) {
navigation.navigate("Home");
}
});
};
const handleRegisterPress = () => {
auth
.createUserWithEmailAndPassword(email, password)
.then((user) => {
console.log(user);
})
.catch((e) => alert(e));
};
return (
<View style={styles.container}>
<View>
<TextInput
value={email}
style={styles.textStyle}
placeholder="Email"
placeholderTextColor="white"
onChangeText={handleChangeEmail}
/>
<TextInput
value={password}
style={styles.textStyle}
placeholder="Password"
placeholderTextColor="white"
onChangeText={handleChangePassword}
/>
</View>
<View>
<TouchableOpacity
style={styles.activateStyle}
onPress={handleLoginPress}
>
<Text style={styles.activeTextStyle}>Login</Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.activateStyle}
onPress={handleRegisterPress}
>
<Text style={styles.activeTextStyle}>Register</Text>
</TouchableOpacity>
</View>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: "center",
},
textStyle: {
backgroundColor: "#1167CC",
width: "80%",
height: 52,
marginVertical: 10,
borderRadius: 26,
alignSelf: "center",
paddingHorizontal: 20,
},
activateStyle: {
backgroundColor: "#1167CC",
marginVertical: 5,
height: 40,
width: "40%",
borderRadius: 26,
alignSelf: "center",
paddingTop: 10,
},
activeTextStyle: {
color: "#ffff",
textAlign: "center",
textAlignVertical: "center",
height: 52,
},
});
Here, in the above code,
Line 1-9 we are importing all the required dependencies.
Line 12-13, we are using useState for the email and password
Line 15-22, we are using useEffect. If the user is already registered then it must navigate to the Home page.
Line 24-29 , Setting Email and Password to useState
Line 30-36, We have created a function called handleLoginPress , where it checks if the user has been registered or not.
Line 37-44, It registers a user in firebase using createUserWithEmailAndPassword
Conclusion
Above, I have a simple way to login/register in firebase. Please feel free to comment.
Here is the link to Github page.