JavaScript Basics for beginners
Javascript is a dynamic language that means -> Datatypes of the variables can change during runtime.
Javascript determines data types depending on the values assigned.We can get datatype by using ‘typeOf’ function.
There are 8 datatypes in Javascript and we can classify these 8 datatypes into 2 categories:
Primitive Data types string,number,null,,undefined,boolean,symbol,bigInt
Object Data types:Object
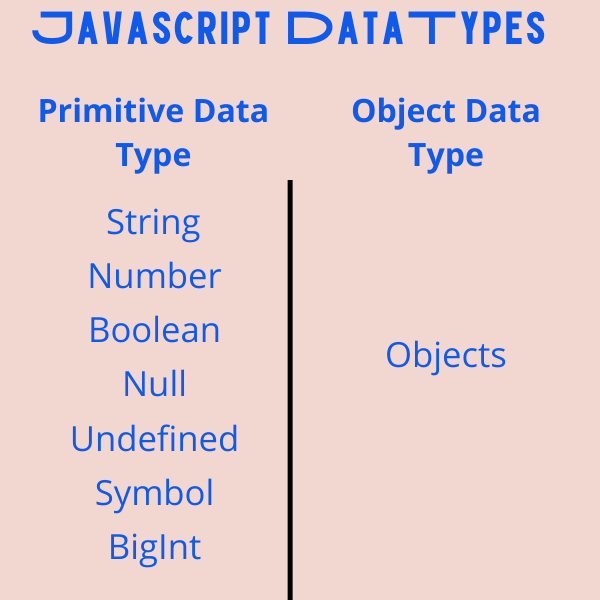
Commonly used datatypes – string, number, null, undefined, boolean, object
Undefined datatypes: it indicates that the variable has been declared but no value is assigned to it.
var a = 1;
var b = 2;
var c;
Null datatypes: indicates absence of data . Its not ZERO, it not empty but just absence of data
var a = 1;
var b = 2;
var c = null;
Null and Undefined Difference:
Undefined : Variable has been created but value is not assigned.
Null: We assign value NULL, it indicated absence of data.
Javascript Hoisting:It’s a mechanism where variables and functions declaration are move to the top of the scope
Global Variables: are variables which are accessible throughout the webpage or the document. What happens when you declare variable with out VAR ?
var x = 10;
function func1() {
y = 100;
console.log(x);
}
func1();
console.log(y);
If we declare the variable without the Var, then the variable becomes global, here y is 100