Conditionals in Javascript
Conditions in any programming language are the control behavior which determines whether or not that piece of code can run.
Some of the conditions used in Javascript are described below
If else
“If” statements: if a condition is true for a block of code
“Else” statements: where if the same condition is false for a block of code
Else if” statements: this specifies a new test if the first condition is false.
var temp = 20
if (temp === 10) {
console.log('Temperature is ok')
}
else if( temp === 0) {
console.lof('Its 0 degree celcius')
}
else {
console.log('Its hot')
}
Output: Its hot
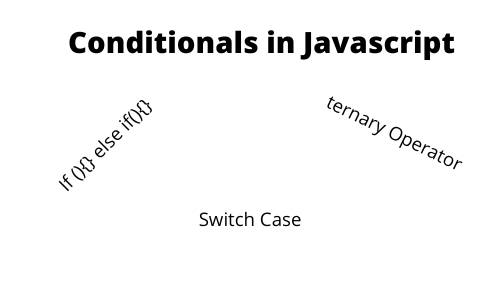
Conditional (ternary) operator
It takes three operands. It has a condition which is followed by a question Mark(?), then followed by the expression to execute if the condition is true and then followed by semiColon(:) which is executed if the condition is false. It is mostly used as alternative to if else condition.
Syntax
condition ? expressionIfTrue : expressionIfFalse
Example
var isSelected = true
isSelected ? console.log('selected true') : console.log('selected false')
The Switch Case
The switch
statement is used to perform different actions based on values of each statement.
syntax
switch(expression) {
case x:
execute code
break;
case y:
execute code
break;
default:
execute code
}
This is how it works:
- The switch expression is evaluated once.
- The value of the expression is compared with the values of each case.
- If there is a match, the associated block of code is executed.
- If there is no match, the default code block is executed.